프로젝트에서 modelMapper.map으로 조회 해온 entity를 응답 dto에 담아서 보내주는 상황을 보고 조금 더 자세히 알아보고 싶어졌다.
class Name {
String name;
}
class Address {
String country;
String postcode;
}
class User {
Name name;
}
class OrderDto {
String custName;
String custCountry;
String custPostCode;
}
Order order = new Order(
new User(new Name("limemint")),
new Address(new Address("kor", "333333"));
);
ModelMapper modelMapper = new ModelMapper();
OrderDto result = modelMapper.map(order, OrderDto.class);
result : custName = "limemint" custCode = "333333" custCountry = "kor"
성공적으로 매핑 된다.
ModelMapper에서 map(source, destination)이 호출 되면 둘의 타입을 분석해서 설정 값에 따라 일치하는 속성을 결정하고 매핑을 진행한다.
프로퍼티들이 서로 일치하는 경우이다
연관 관계가 모호하면 매핑이 원하는 방향으로 진행 되지 않을 수도 있다. 이럴때는 설정 값을 통해서 mapping 해보자
Type Map
package example;
public class Bill {
private String itemName;
private Integer nnn;
private Integer someThingPrice;
public Bill() {
}
public Bill(String itemName, Integer nnn, Integer someThingPrice) {
this.itemName = itemName;
this.nnn = nnn;
this.someThingPrice = someThingPrice;
}
public String getItemName() {
return itemName;
}
public void setItemName(String itemName) {
this.itemName = itemName;
}
public Integer getNnn() {
return nnn;
}
public void setNnn(Integer nnn) {
this.nnn = nnn;
}
public Integer getSomeThingPrice() {
return someThingPrice;
}
public void setSomeThingPrice(Integer someThingPrice) {
this.someThingPrice = someThingPrice;
}
}
package example;
public class Item {
private String name;
private Integer price;
private Integer stock;
public Item() {
}
public Item(String name, Integer price, Integer stock) {
this.name = name;
this.price = price;
this.stock = stock;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getPrice() {
return price;
}
public void setPrice(Integer price) {
this.price = price;
}
public Integer getStock() {
return stock;
}
public void setStock(Integer stock) {
this.stock = stock;
}
}
package example;
import org.modelmapper.ModelMapper;
public class Example {
public static void main(String[] args) {
ModelMapper modelMapper = new ModelMapper();
Item item = new Item("item", 1500, 123);
Bill bill = modelMapper.map(item, Bill.class);
}
}
결과
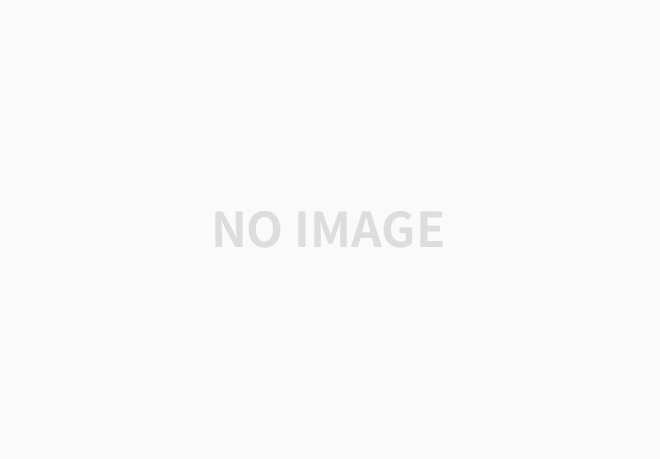
위와 같은 모호한 연관 관계들은 매핑이 안된 상황이다
이런 문제 해결을 위해 TypeMap<S,D> 를 사용해보자!
item.stock - bill.nnn
item.price - bill.someThingPrice 와 매핑 되길 원한다.
package example;
import org.modelmapper.ModelMapper;
public class Example {
public static void main(String[] args) {
ModelMapper modelMapper = new ModelMapper();
Item item = new Item("item", 1500, 123);
modelMapper.typeMap(Item.class, Bill.class).addMappings(
mapper -> {
mapper.map(Item::getStock, Bill ::setNnn);
mapper.map(Item::getPrice, Bill::setSomeThingPrice);
});
Bill bill2 = modelMapper.map(item, Bill.class);
}
}
결과 :
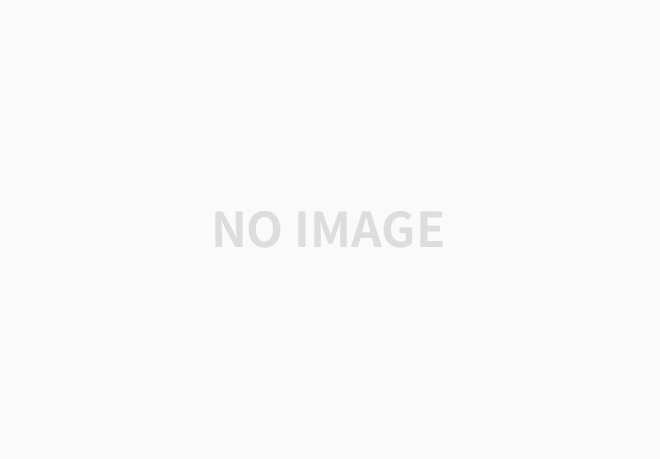
매핑 성공이다!
현재 사용하는건 이정도라서 다른 방식 정리는 나중에 추가하겠다!
참고 :
http://modelmapper.org/user-manual/
ModelMapper - User Manual
User Manual The goal of the user manual is to provide an overview of ModelMapper’s features along with details about how it works. If you’re new to ModelMapper, check out the Getting Started guide first. To browser the User Manual, choose from the menu
modelmapper.org
'개발 > JAVA' 카테고리의 다른 글
모던 자바 인 액션 스터디 (0) | 2022.10.13 |
---|---|
@NoArgsConstructor(access = PROTECTED)에 관하여 (1) | 2022.09.12 |
Iterator (0) | 2022.07.11 |
자바 8 정리 (0) | 2022.07.08 |
Junit - Assert 메소드 (1) | 2022.06.02 |